This article is a guide to creating a (MSTest) test project for a JWT secured ASP.NET Core Web API. Where both the Web API under test and the tests themselves are using the VSC.App.Core.WebApi library.
For details about how to create a Web API using the VSC.App.Core.WebApi library, see here.
Start with a standard MSTest test project. Add the VSC.App.Core.WebApi project. This provides:
- the web data model for JWT sign in as well;
- some standard base classes for response web data models;
- an AuthenticateController to add JWT sign in to your API;
- a TestController that provides methods to test the authentication.
Now add the VSC.App.Core.WebApi.Test project.
You will need to add a config.json file to the core test project to match the Web API under test. This must include the base URL for the Web API under test, and sign-in details for an account that can be used to test authentication. For example:
{
"urlBase": "https://localhost:7120",
"userName": "test@test.com",
"password": "Password99!"
}
Make sure your config.json build action is set to ‘Copy always’.
This gives you the ability to test the authentication part of the Web API under test.
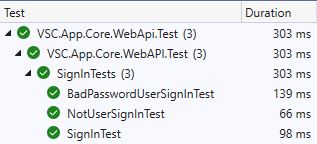
These tests are based on some standard Web API methods that are added to your Web API when it is based on the VSC.App.Core.WebApi project.
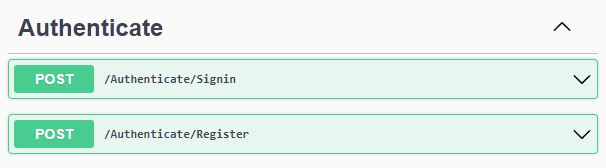
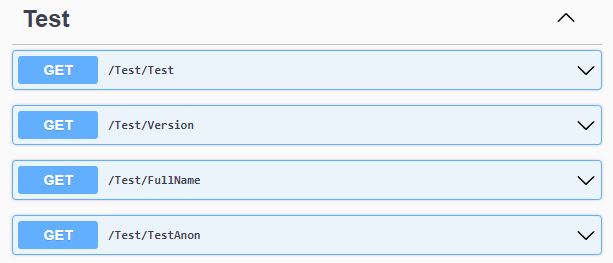
Now add your own test classes and methods for the Web API under test.
Base your test classes on the WebApiTestSecuredJwt class, and add a config.json file (as before). For example:
[TestClass]
public class UnitTest1 : WebApiTestSecuredJwt
{
[TestMethod]
public async Task TestMethod1()
{
await SignIn();
}
}
The test run will sign in using the credentials in the config.json file:

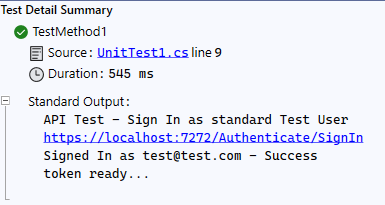
The WebApiTestSecuredJwt base class provides the GetBasedUri helper which reads the base URL from the config.json file and adds a relative path. It also provides the HttpClient object, which after sign-in, will have its security headers populated with a valid JWT as a ‘Bearer Token’.
So after sign-in, the test code can proceed with assembling the URL and creating the request, before sending the request and receiving the response. For example:
...
var uri = GetBasedUri("/DoThis");
var payload = new DoThisRequest {FirstName = "John", LastName = "Doe"};
var request = new HttpRequestMessage(HttpMethod.Post, uri)
{
Content = new StringContent(JsonConvert.SerializeObject(payload),
Encoding.UTF8, "application/json")
};
var response = await HttpClient.SendAsync(request);
if (response.IsSuccessStatusCode)
{
var model = await response.Content.ReadAsAsync<MyWebDataModel>();
...
Further, as we base our web data models on standard base classes for the response, more information will be available on the status of the model retrieved. See here for more information.